Aliexpress contains millions of products and product reviews that can be used in market analytics, business intelligence and dropshipping.
In this tutorial, we'll take a look at how to scrape Aliexpress. We'll start by finding products by scraping the search system. Then we'll scrape the found product data, pricing and customer reviews.
This will be a relatively easy scraper in just a few lines of Python code. Let's dive in!
Latest Aliexpress.com Scraper Code
Legal Disclaimer and Precautions
This tutorial covers popular web scraping techniques for education. Interacting with public servers requires diligence and respect and here's a good summary of what not to do:- Do not scrape at rates that could damage the website.
- Do not scrape data that's not available publicly.
- Do not store PII of EU citizens who are protected by GDPR.
- Do not repurpose the entire public datasets which can be illegal in some countries.
Why Scrape Aliexpress?
There are many reasons to scrape Aliexpress data. For starters, because Aliexpress is the biggest e-commerce platform in the world, it's a prime target for business intelligence or market analytics. Having an awareness of top products and their meta-information on Aliexpress can be used to great advantage in business and market analysis.
Another common use is e-commerce primarily via dropshipping - one of the biggest emergent markets of this century is curating a list of products and reselling them directly rather than managing a warehouse. In this case, many shop curators would scrape Aliexpress products to generate curated product lists for their dropshipping shops.
Project Setup
In this tutorial we'll be using Python with two packages:
- httpx - HTTP client library, which will let us communicate with AliExpress.com's servers
- parsel - HTML parsing library, which will help us to parse our web scraped HTML files for product data.
- jmespath - JSON parsing library, which we'll use to refine very long JSON datasets.
All of these packages can be easily installed via pip
command:
$ pip install httpx parsel jmespath
Alternatively, you're free to swap httpx
out with any other HTTP client library such as requests as we'll only need basic HTTP functions which are almost interchangeable in every library. As for, parsel
, another great alternative is beautifulsoup package.
Web Scraping with Python
Introduction tutorial to web scraping with Python. How to collect and parse public data. Challenges, best practices and an example project.
Finding Aliexpress Products
There are many ways to discover products on Aliexpress.
We could use the search system to find products we want to scrape or explore many product categories. Whichever approach we take our key target is all the same - scrape product previews and pagination.
Let's take a look at Aliexpress listing page that is used in the search or category view:
If we take a look at the page source of either search or category page we can see that all the product previews are stored in a javascript variable window.runParams
tucked away in the <script>
tag in the HTML source of the page:
This is a common web development pattern, which enables dynamic data management using javascript.
It's good news for us though, as we can pick this data up with a simple regex pattern and parse it like a Python dictionary! This is generally called hidden web data scraping and it's a common pattern in modern web scraping.
With this, we can write the first piece of our Aliexpress scraper code - the product preview parser. We'll be using it to extract product preview data from category or search result pages:
from parsel import Selector
from typing import Dict
import httpx
import json
def extract_search(response) -> Dict:
"""extract json data from search page"""
sel = Selector(response.text)
# find script with result.pagectore data in it._it_t_=
script_with_data = sel.xpath('//script[contains(.,"_init_data_=")]')
# select page data from javascript variable in script tag using regex
data = json.loads(script_with_data.re(r'_init_data_\s*=\s*{\s*data:\s*({.+}) }')[0])
return data['data']['root']['fields']
def parse_search(response):
"""Parse search page response for product preview results"""
data = extract_search(response)
parsed = []
for result in data["mods"]["itemList"]["content"]:
parsed.append(
{
"id": result["productId"],
"url": f"https://www.aliexpress.com/item/{result['productId']}.html",
"type": result["productType"], # can be either natural or ad
"title": result["title"]["displayTitle"],
"price": result["prices"]["salePrice"]["minPrice"],
"currency": result["prices"]["salePrice"]["currencyCode"],
"trade": result.get("trade", {}).get("tradeDesc"), # trade line is not always present
"thumbnail": result["image"]["imgUrl"].lstrip("/"),
"store": {
"url": result["store"]["storeUrl"],
"name": result["store"]["storeName"],
"id": result["store"]["storeId"],
"ali_id": result["store"]["aliMemberId"],
},
}
)
return parsed
Let's try our parser out by scraping a single Aliexpress listing page (category page or search results page):
Run code & example output
if __name__ == "__main__":
# for example, this category is for android phones:
resp = httpx.get("https://www.aliexpress.com/category/5090301/cellphones.html", follow_redirects=True)
print(json.dumps(parse_search(resp), indent=2, ensure_ascii=False))
[
{
"id": "3256804075561256",
"url": "https://www.aliexpress.com/item/3256804075561256.html",
"type": "ad",
"title": "2G/3G Smartphones Original 512MB RAM/1G RAM 4GB ROM android mobile phones new cheap celulares FM unlocked 4.0inch cell",
"price": 21.99,
"currency": "USD",
"trade": "8 sold",
"thumbnail": "ae01.alicdn.com/kf/S1317aeee4a064fad8810a58959c3027dm/2G-3G-Smartphones-Original-512MB-RAM-1G-RAM-4GB-ROM-android-mobile-phones-new-cheap-celulares.jpg_220x220xz.jpg",
"store": {
"url": "www.aliexpress.com/store/1101690689",
"name": "New 123 Store",
"id": 1101690689,
"ali_id": 247497658
}
}
...
]
There's a lot of useful information, but we've limited our parser to bare essentials to keep things brief. Next, let's put this parser to use in actual scraping.
Scraping Aliexpress Search
Now that we have our product preview parser ready, we need a scraper loop that will iterate through search results to collect all available results - not just the first page:
from parsel import Selector
from typing import Dict
import httpx
import math
import json
import asyncio
def extract_search(response) -> Dict:
# rest of the function logic
def parse_search(response):
# rest of the function logic
async def scrape_search(query: str, session: httpx.AsyncClient, sort_type="default", max_pages: int = None):
"""Scrape all search results and return parsed search result data"""
query = query.replace(" ", "+")
async def scrape_search_page(page):
"""Scrape a single aliexpress search page and return all embedded JSON search data"""
print(f"scraping search query {query}:{page} sorted by {sort_type}")
resp = await session.get(
"https://www.aliexpress.com/wholesale?trafficChannel=main"
f"&d=y&CatId=0&SearchText={query}<ype=wholesale&SortType={sort_type}&page={page}"
)
return resp
# scrape first search page and find total result count
first_page = await scrape_search_page(1)
first_page_data = extract_search(first_page)
page_size = first_page_data["pageInfo"]["pageSize"]
total_pages = int(math.ceil(first_page_data["pageInfo"]["totalResults"] / page_size))
if total_pages > 60:
print(f"query has {total_pages}; lowering to max allowed 60 pages")
total_pages = 60
# get the number of total pages to scrape
if max_pages and max_pages < total_pages:
total_pages = max_pages
# scrape remaining pages concurrently
print(f'scraping search "{query}" of total {total_pages} sorted by {sort_type}')
other_pages = await asyncio.gather(*[scrape_search_page(page=i) for i in range(1, total_pages + 1)])
for response in [first_page, *other_pages]:
product_previews = []
product_previews.extend(parse_search(response))
return product_previews
Run the code
async def run():
client = httpx.AsyncClient(follow_redirects=True)
data = await scrape_search(query="cell phones", session=client, max_pages=3)
print(json.dumps(data, indent=2, ensure_ascii=False))
if __name__ == "__main__":
asyncio.run(run())
Above, we defined our scrape_search
function we use a common web scraping idiom for known length pagination:
We scrape the first page to extract the total number of pages and scrape the remaining pages concurrently. We also add a max_pages
parameter to control the number of pagination pages.
Now, that we can find products let's take a look at how we can scrape product data, pricing info and reviews!
Scraping Aliexpress Products
Aliexpress product pages are protected againist CAPTCHA challenges and load its data using JavaScript. Hence, scraping Aliexpress product pages requires JavaScript to be enabled. For this, we'll use ScrapFly's JavaScript rendering feature.
To scrape Aliexpress products. all we need is a product numeric ID, which we already found in the previous chapter by scraping product previews from the Aliexpress search scraper. For example, this hand drill product has the numeric ID of 4000927436411.html .
We'll request the product pages and parse their data using XPath selectors:
import json
import asyncio
from typing import Dict
from scrapfly import ScrapeApiResponse, ScrapeConfig, ScrapflyClient, ScrapflyScrapeError
BASE_CONFIG = {
"asp": True,
"country": "US",
# aliexpress returns differnt results based on localization settings
# apply localization settings from the browser and then copy the aep_usuc_f cookie from devtools
"headers": {
"cookie": "aep_usuc_f=site=glo&province=&city=&c_tp=USD®ion=EG&b_locale=en_US&ae_u_p_s=2"
}
}
SCRAPFLY = ScrapflyClient(key="Your ScrapFly API key")
def parse_product(result: ScrapeApiResponse) -> Product:
"""parse product HTML page for product data"""
selector = result.selector
reviews = selector.xpath("//a[contains(@class,'reviewer--reviews')]/text()").get()
rate = selector.xpath("//div[contains(@class,'rating--wrap')]/div").getall()
sold_count = selector.xpath("//span[contains(@class,'reviewer--sold')]/text()").get()
available_count = selector.xpath("//div[contains(@class,'quantity--info')]/div/span/text()").get()
info = {
"name": selector.xpath("//h1[@data-pl]/text()").get(),
"productId": int(result.context["url"].split("item/")[-1].split(".")[0]),
"link": result.context["url"],
"media": selector.xpath("//div[contains(@class,'slider--img')]/img/@src").getall(),
"rate": len(rate) if rate else None,
"reviews": int(reviews.replace(" Reviews", "")) if reviews else None,
"soldCount": int(sold_count.replace(" sold", "").replace(",", "").replace("+", "")) if sold_count else None,
"availableCount": int(available_count.replace(" available", "")) if available_count else None
}
price = selector.xpath("//span[contains(@class,'currentPrice')]/text()").get()
original_price = selector.xpath("//span[contains(@class,'price--originalText')]/text()").get()
discount = selector.xpath("//span[contains(@class,'price--discount')]/text()").get()
pricing = {
"priceCurrency": "USD $",
"price": float(price.split("$")[-1]) if price else None, # for US localization
"originalPrice": float(original_price.split("$")[-1]) if price else "No discount",
"discount": discount if discount else "No discount",
}
shipping_cost = selector.xpath("//strong[contains(text(),'Shipping')]/text()").get()
shipping = {
"cost": float(shipping_cost.split("$")[-1]) if shipping_cost else None,
"currency": "$",
"delivery": selector.xpath("(//div[contains(@class,'dynamic-shipping-line')])[2]/span[2]/span/strong/text()").get()
}
specifications = []
for i in selector.xpath("//div[contains(@class,'specification--prop')]"):
specifications.append({
"name": i.xpath(".//div[contains(@class,'specification--title')]/span/text()").get(),
"value": i.xpath(".//div[contains(@class,'specification--desc')]/span/text()").get()
})
faqs = []
for i in selector.xpath("//div[@class='ask-list']/ul/li"):
faqs.append({
"question": i.xpath(".//p[@class='ask-content']/text()").get(),
"answer": i.xpath(".//ul[@class='answer-box']/li/p/text()").get()
})
seller_link = selector.xpath("//a[@data-pl='store-name']/@href").get()
seller_followers = selector.xpath("//div[contains(@class,'store-info')]/strong[2]/text()").get()
seller_followers = int(float(seller_followers.replace('K', '')) * 1000) if seller_followers and 'K' in seller_followers else int(seller_followers) if seller_followers else None
seller = {
"name": selector.xpath("//a[@data-pl='store-name']/text()").get(),
"link": seller_link.split("?")[0].replace("//", "") if seller_link else None,
"id": int(seller_link.split("store/")[-1].split("?")[0]) if seller_link else None,
"info": {
"positiveFeedback": selector.xpath("//div[contains(@class,'store-info')]/strong/text()").get(),
"followers": seller_followers
}
}
return {
"info": info,
"pricing": pricing,
"specifications": specifications,
"shipping": shipping,
"faqs": faqs,
"seller": seller,
}
async def scrape_product(url: str) -> Dict:
"""scrape aliexpress products by id"""
print("scraping product: {}", url)
result = await SCRAPFLY.async_scrape(ScrapeConfig(
url, **BASE_CONFIG, render_js=True, session=session_id, auto_scroll=True,
rendering_wait=15000, retry=False, timeout=150000, js_scenario=[
{"wait_for_selector": {"selector": "//div[@id='nav-specification']//button", "timeout": 5000}},
{"click": {"selector": "//div[@id='nav-specification']//button", "ignore_if_not_visible": True}}
]
))
data = parse_product(result)
print("successfully scraped product: {}", url)
return data
Run the code
async def run():
data = await scrape_product(
url="https://www.aliexpress.com/item/1005006717259012.html"
)
with open("product.json", "w", encoding="utf-8") as file:
json.dump(data, file, indent=2, ensure_ascii=False)
if __name__ == "__main__":
asyncio.run(run())
Here, wait for the full page to load and click the button responsible for loading the full product specifications. Then, we use XPath selectors to parse the full product details. Here's what the retrieved results should look like:
Eexample output
{
"info": {
"name": "10mm Electric Brushless Drill 2-Speed Self-locking Cordless Drill Screwdriver 60-100Nm Torque Power Tools For Makita 18V Battery",
"productId": 1005006717259012,
"link": "https://www.aliexpress.com/item/1005006717259012.html",
"media": [
"https://ae-pic-a1.aliexpress-media.com/kf/Sab4cf830d63149b7acf4b95773a75fe2k.png_80x80.png_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/S8129eebce8fd466993f36afd1e874563Z.jpg_80x80.jpg_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/Sa5c6d11d7bf54b98b756438067f08c25S.png_80x80.png_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/Sf865a7763bda4c1c9366b6c91763df922.png_80x80.png_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/Sb0f9bd88a59e4c859acb21e1b48e821e4.png_80x80.png_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/S03f54a829e464bfbafc5df0741d5007d4.jpg_80x80.jpg_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/S41bbac0e2a2a4bfd87bba0307e69a040G.jpg_120x120.jpg_.webp",
"https://ae-pic-a1.aliexpress-media.com/kf/S0cb3b457c92d4e82825365d4b1bfc66ac.png_120x120.png_.webp"
],
"rate": 5,
"reviews": 494,
"soldCount": 2000,
"availableCount": null
},
"pricing": {
"priceCurrency": "USD $",
"price": 40.04,
"originalPrice": 42.8,
"discount": "6% off"
},
"specifications": [
{
"name": "Hign-concerned Chemical",
"value": "None"
},
{
"name": "Max. Drilling Diameter",
"value": "10mm"
},
{
"name": "Origin",
"value": "Mainland China"
},
{
"name": "Brand Name",
"value": "PATUOPRO"
},
{
"name": "Motor type",
"value": "Brushless"
},
{
"name": "Power Source",
"value": "Battery"
},
{
"name": "Drill Type",
"value": "Cordless Drill"
},
{
"name": "No Load Speed",
"value": "0-450/0-2000r/min"
},
{
"name": "Torque Setting",
"value": "21+1"
},
{
"name": "Chuck Size",
"value": "3/8\" (0.8-10mm)"
}
],
"shipping": {
"cost": null,
"currency": "$",
"delivery": "Oct 04"
},
"faqs": [
{
"question": "Is the device as powerful as the observed force on the chord known by DTD",
"answer": null
},
{
"question": "Comes with charger",
"answer": null
},
{
"question": "Bought a patuopro screwdriver jammed the engine. I can't find the same on the site. 50 cm long",
"answer": null
}
],
"seller": {
"name": "PATUOPRO Official Store",
"link": "//www.aliexpress.com/store/1102818328",
"id": 1102818328,
"info": {
"positiveFeedback": "97.2%",
"followers": "2292"
}
}
}
Above, we extracted the full product details. However, we miss the review data themselves. Let's take a look at how we can retrieve the review data.
Scraping Aliexpress Reviews
Aliexpress' product reviews are dynamically retrieved through background API requests. To view this API, follow the below steps:
- Open the browser developer tools by pressing the
F12
key - Select the Network tab and filter by
Fetch/XHR
calls - Trigger the review API by clicking the "View More" button to fetch all reviews
After following the above steps, you will the below XHR request captured:
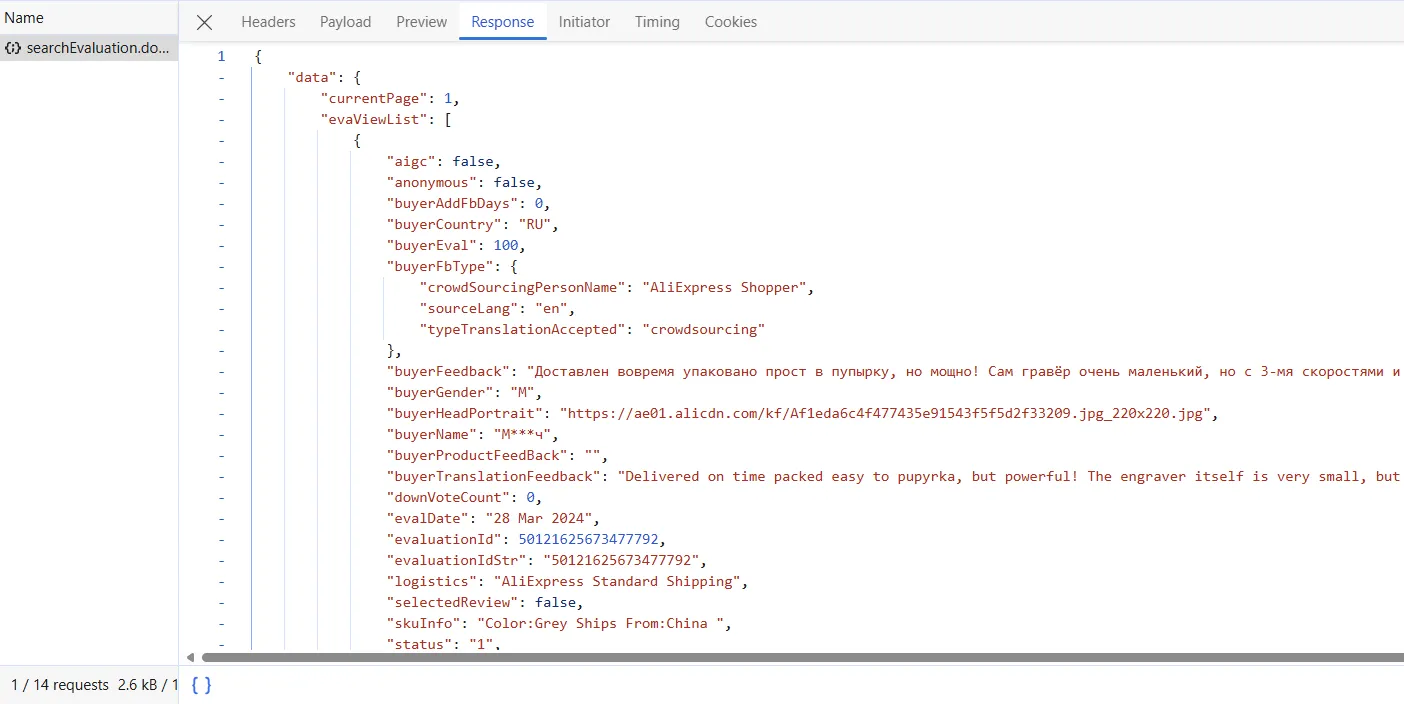
Above, we can see the review data retrieved directly as JSON, which is later rendered into HTML. To scrape Aliexpress reviews, we'll request the API endpoint directly. This approach is commonly known as hidden API scraping.
How to Scrape Hidden APIs
In this tutorial we'll be taking a look at scraping hidden APIs which are becoming more and more common in modern dynamic websites - what's the best way to scrape them?
Let's request the hidden Aliexpress reviews API within our scraper. We'll also utilize its URL parameters for pagination:
import json
import asyncio
from httpx import AsyncClient
client = AsyncClient(
# enable http2
http2=True,
# add basic browser like headers to prevent getting blocked
headers={
"Accept-Language": "en-US,en;q=0.9",
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/96.0.4664.110 Safari/537.36",
"Accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8",
"Accept-Encoding": "gzip, deflate, br",
}
)
def parse_review_page(response):
data = json.loads(response.text)["data"]
return {
"max_pages": data["totalPage"],
"reviews": data["evaViewList"],
"evaluation_stats": data["productEvaluationStatistic"]
}
async def scrape_product_reviews(product_id: str, max_scrape_pages: int = None):
"""scrape all reviews of aliexpress product"""
def scrape_config_for_page(page):
url = f"https://feedback.aliexpress.com/pc/searchEvaluation.do?productId={product_id}&lang=en_US&country=US&page={page}&pageSize=10&filter=all&sort=complex_default"
return url
# scrape first page of reviews and find total count of review pages
first_page_result = await client.get(scrape_config_for_page(1))
data = parse_review_page(first_page_result)
max_pages = data["max_pages"]
if max_scrape_pages and max_scrape_pages < max_pages:
max_pages = max_scrape_pages
# create scrape configs for other pages and scrape them concurrently
print(f"scraping reviews pagination of product {product_id}, {max_pages - 1} pages remaining")
to_scrape = [client.get(scrape_config_for_page(page)) for page in range(2, max_pages + 1)]
for response in asyncio.as_completed(to_scrape):
response = await response
data["reviews"].extend(parse_review_page(response)["reviews"])
print(f"scraped {len(data["reviews"])} from review pages")
data.pop("max_pages")
return data
Run the code
async def run():
review_results = await scrape_product_reviews(
product_id="1005006717259012",
max_scrape_pages=3
)
# save the results to a JSON file
with open("reviews.json", "w", encoding="utf-8") as file:
json.dump(review_results, file, indent=2, ensure_ascii=False)
if __name__ == "__main__":
asyncio.run(run())
Above, we create a review crawler. It starts by requesting the review API for the first page to retrieve the total number of pages available. Then, the remaining review pages are sccraped concurrently. Here's an example output of the above Aliexpress scraper:
Example Output
{
"reviews": [
{
"aigc": false,
"anonymous": false,
"buyerAddFbDays": 0,
"buyerCountry": "US",
"buyerEval": 80,
"buyerFbType": {
"crowdSourcingPersonName": "AliExpress Shopper",
"sourceLang": "en",
"typeTranslationAccepted": "crowdsourcing"
},
"buyerFeedback": "Патрон меьалевий, все інше пластик, дуже легкий, патрон не швидкознімний - для дому піде, дуууже легкий.",
"buyerName": "a***n",
"buyerProductFeedBack": "",
"buyerTranslationFeedback": "The cartridge is mealevy, everything is plastic, it is light, the cartridge is not shvidkoznym-for the House, the duuguge is light.",
"downVoteCount": 0,
"evalDate": "25 Apr 2024",
"evaluationId": 30072011607750988,
"evaluationIdStr": "30072011607750988",
"logistics": "Aliexpress Selection Standard",
"selectedReview": false,
"skuInfo": "Color:1PC 2.0Ah Battery Plug Type:EU Ships From:CHINA ",
"status": "1",
"trendyol": false,
"upVoteCount": 0
},
....
],
"evaluation_stats": {
"evarageStar": 4.8,
"evarageStarRage": 96.8,
"fiveStarNum": 447,
"fiveStarRate": 89.0,
"fourStarNum": 31,
"fourStarRate": 6.2,
"negativeNum": 15,
"negativeRate": 3.0,
"neutralNum": 9,
"neutralRate": 1.8,
"oneStarNum": 11,
"oneStarRate": 2.2,
"positiveNum": 478,
"positiveRate": 95.2,
"threeStarNum": 9,
"threeStarRate": 1.8,
"totalNum": 502,
"twoStarNum": 4,
"twoStarRate": 0.8
}
With this last feature, we've covered the main scrape targets of Aliexpress - we scraped search to find products, product pages to find product data and product reviews to gather feedback intelligence. Finally, to scrape at scale let's take a look at how can we avoid blocking and captchas.
Bypass Aliexpress Blocking and Captchas
Scraping product data of Aliexpress.com seems to be easy though unfortunately when scraping at the scale we might be blocked or requested to start solving captchas which will hinder our web scraping process.
To get around this, let's take advantage of ScrapFly API, which can avoid all of these blocks for us!
Which offers several powerful features that'll help us to get around AliExpress's blocking:
For this, we'll be using scrapfly-sdk python package and ScrapFly's anti scraping protection bypass feature. First, let's install scrapfly-sdk
using pip:
$ pip install scrapfly-sdk
To take advantage of ScrapFly's API in our AliExpress product scraper all we need to do is our httpx
session code with scrapfly-sdk
requests.
FAQ
To wrap this guide up, let's take a look at some frequently asked questions about web scraping aliexpress.com:
Is web scraping aliexpress.com legal?
Yes. Aliexpress product data is publicly available, and we're not extracting anything personal or private. Scraping aliexpress.com at slow, respectful rates would fall under the ethical scraping definition. See our Is Web Scraping Legal? article for more.
Is there an Aliexpress API?
No. Currently there's no public API for retrieving product data from Aliexpress.com. Fortunately, as covered in this tutorial, web scraping Aliexpress is easy and can be done with a few lines of Python code!
Scraped Aliexpress data is not accurate, what can I do?
The main cause of data difference is geo location. Aliexpress.com shows different prices and products based on the user's location so the scraper needs to match the location of the desired data. See our previous guide on web scraping localization for more details on changing the web scraping language, price or location.
Aliexpress Scraping Summary
In this tutorial, we built an Aliexpress data scraper capable of using the search system to discover products and scraping product data and product reviews.
We have used Python with httpx and parsel packages and to avoid being blocked we used ScrapFly's API, which smartly configures every web scraper connection to avoid being blocked. For more on ScrapFly see our documentation and try it out for free!